How to Print a MapBoxGL.js Map to a PDF in React
Blog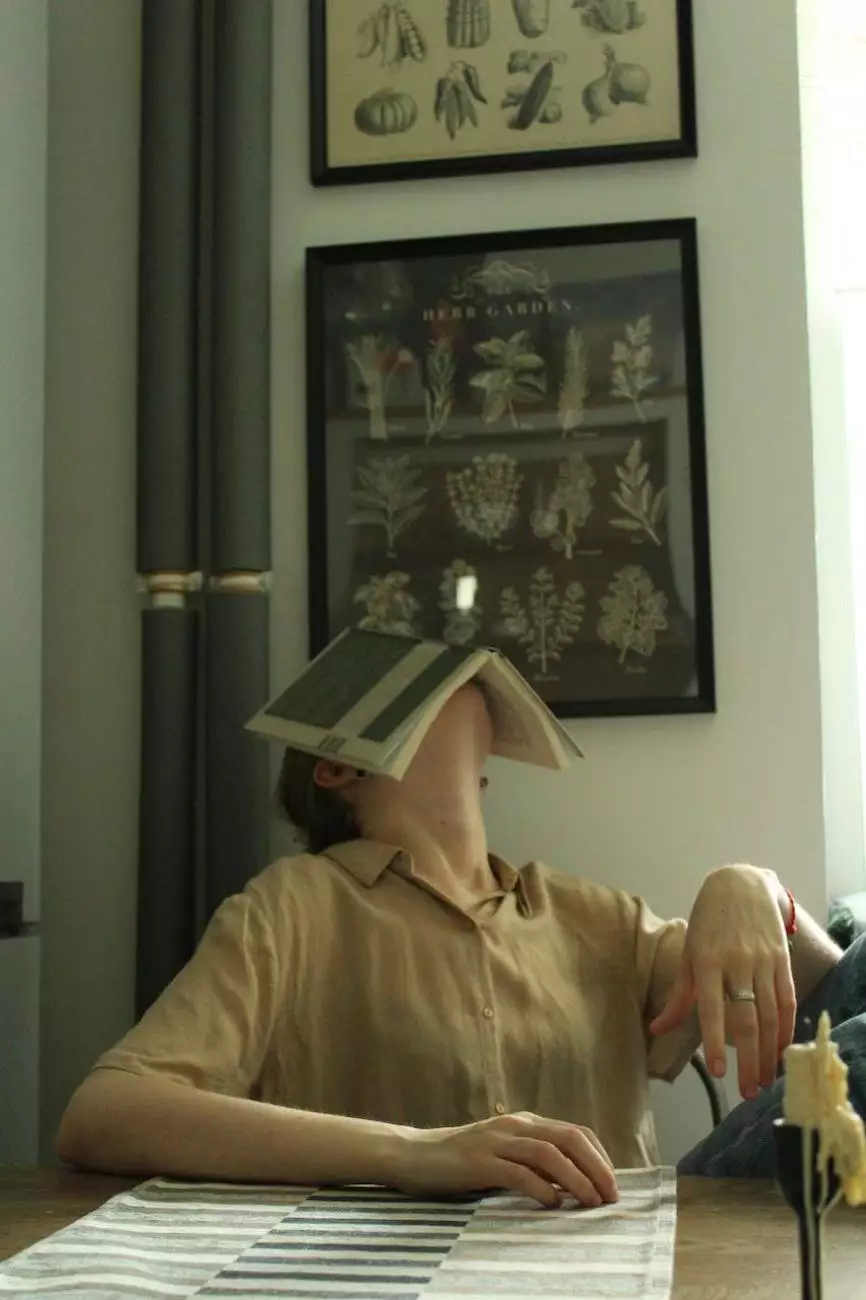
Welcome to the comprehensive guide on how to print a MapBoxGL.js map to a PDF in React, brought to you by Newark SEO Experts, a top-tier digital marketing agency specializing in Business and Consumer Services. In this in-depth tutorial, we will walk you through the process step by step, providing you with all the necessary details and code snippets to successfully accomplish this task.
Understanding MapBoxGL.js and React
Before diving into the process of printing a MapBoxGL.js map to a PDF in React, it is important to have a basic understanding of these two technologies.
MapBoxGL.js is a powerful JavaScript library that allows you to create interactive, customizable maps for your applications. It offers a wide range of features and functionalities, making it a popular choice among developers.
React, on the other hand, is a JavaScript library for building user interfaces. It provides a component-based approach to web development, making it easy to create reusable UI components that can update efficiently and dynamically.
Step 1: Setting up the Development Environment
Before we start coding, let's ensure that we have the necessary tools and dependencies installed in our development environment.
First, make sure you have Node.js and npm (Node Package Manager) installed. You can download and install them from the official Node.js website.
Once Node.js and npm are installed, open your command line interface and run the following command to create a new React project:
npx create-react-app map-to-pdfThis command will create a new directory named "map-to-pdf" with a basic React project structure.
Step 2: Installing MapBoxGL.js
In order to use MapBoxGL.js in our React project, we need to install the required dependencies. Open your command line interface, navigate to the project directory, and run the following command:
npm install mapbox-glThis command will install the MapBoxGL.js library and its dependencies into your project.
Step 3: Creating a Map Component
Now that we have our React project set up and the MapBoxGL.js library installed, let's create a new component to render the map.
Inside the "src" directory of your project, create a new file named "Map.js". Open the file and add the following code:
{ const mapContainer = useRef(null); const [map, setMap] = useState(null); useEffect(() => { mapboxgl.accessToken = 'YOUR_MAPBOX_ACCESS_TOKEN'; const initializeMap = ({ setMap, mapContainer }) => { const mapInstance =new mapboxgl.Map({ container: mapContainer.current, style: 'mapbox://styles/mapbox/streets-v11', center: [longitude, latitude], zoom: zoomLevel }); setMap(mapInstance); }; if (!map) initializeMap({ setMap, mapContainer }); }, [map]); return; }; export default Map;This code defines a functional component named "Map" which renders a element as the container for the map. It initializes the map with the provided longitude, latitude, and zoom level.
Step 4: Rendering the Map Component
Now that we have our Map component defined, let's render it in our main App component.
Open the "src/App.js" file and replace the existing code with the following:
{ const options = { margin: 0, filename: 'map.pdf', image: { type: 'jpeg', quality: 0.98 }, html2canvas: { scale: 2 }, jsPDF: { unit: 'mm', format: 'letter', orientation: 'portrait' } }; html2pdf().set(options).from(mapContainer.current).save(); }; return ( Print Map to PDF ); }; export default Map;In this modified code, we import the html2pdf library and define a new function called "handlePrint". This function is triggered when the "Print Map to PDF" button is clicked. It creates a PDF file containing the map by capturing the rendered HTML using html2canvas, converting it to a PDF using jsPDF, and saving it with the specified filename.
Step 6: Testing and Finalizing
With all the necessary code in place, we can now test our application to see if it successfully prints the MapBoxGL.js map to a PDF in React.
Start your development server by running the following command in your command line interface:
npm startOnce the server is running, open your web browser and visit http://localhost:3000. You should see the "How to Print a MapBoxGL.js Map to a PDF in React" title, a button labeled "Print Map to PDF," and the map rendered on the page.
Click the "Print Map to PDF" button, and a PDF file named "map.pdf" should be downloaded. Open the file to confirm that the map has been successfully printed to PDF.
Conclusion
Congratulations! You have successfully learned how to print a MapBoxGL.js map to a PDF in React. This comprehensive guide provided you with all the necessary steps and code snippets to accomplish this task. Whether you are a developer or a business owner, this knowledge can be useful in various applications and scenarios.
If you have any further questions or encounter any issues along the way, feel free to reach out to Newark SEO Experts, a leading digital marketing agency specializing in Business and Consumer Services. Our team of experts is always available to assist you with your digital marketing needs.
Thank you for choosing Newark SEO Experts, your trusted partner in digital marketing!